Add branch name to commit messages
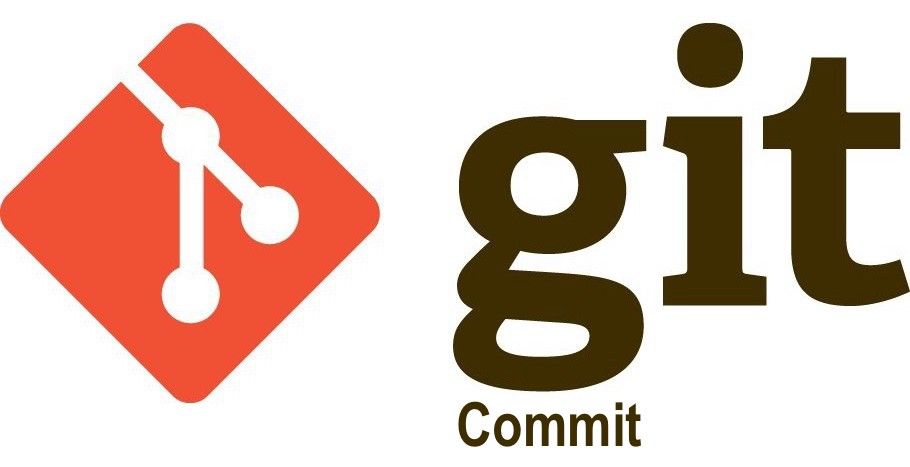
Let's face it. we have to do some "paper pushing" as a developer sometimes so that all the company's tools work together.
USE CASE:
As a developer, we are required to add the JIRA ticket number to our commit messages so that the Product Owner and Stakeholders can see what issues have been completed, and what environment they have been deployed on. JIRA and Bamboo will parse your commit messages for the issues automatically and can assist with this.
ASSUMPTIONS:
You are using git flow
to name your feature branches in a sane way, which includes the JIRA story number in the branch name.
THE PROCESS:
We are going to create a prepare-commit-msg
hook that will do all the work for us.
THE CODE:
#!/bin/bash
# This way you can customize which branches should be skipped when
# prepending commit message.
if [ -z "$BRANCHES_TO_SKIP" ]; then
BRANCHES_TO_SKIP=(master develop test)
fi
BRANCH_NAME=$(git symbolic-ref --short HEAD)
BRANCH_NAME="${BRANCH_NAME##*/}"
BRANCH_EXCLUDED=$(printf "%s\n" "${BRANCHES_TO_SKIP[@]}" | grep -c "^$BRANCH_NAME$")
BRANCH_IN_COMMIT=$(grep -c "\[$BRANCH_NAME\]" $1)
if [ -n "$BRANCH_NAME" ] && ! [[ $BRANCH_EXCLUDED -eq 1 ]] && ! [[ $BRANCH_IN_COMMIT -ge 1 ]]; then
sed -i.bak -e "1s/^/[$BRANCH_NAME] /" $1
fi
IMPLEMENT:
By putting the file prepare-commit-msg
file in the .git/hooks/
directory of your project, you will be good to go.
TROUBLESHOOTING:
You will need to give the prepare-commit-msg
execute permissions or it wont run.
chmod +x .git/hooks/prepare-commit-msg