jQuery animated skill bars for your web resume
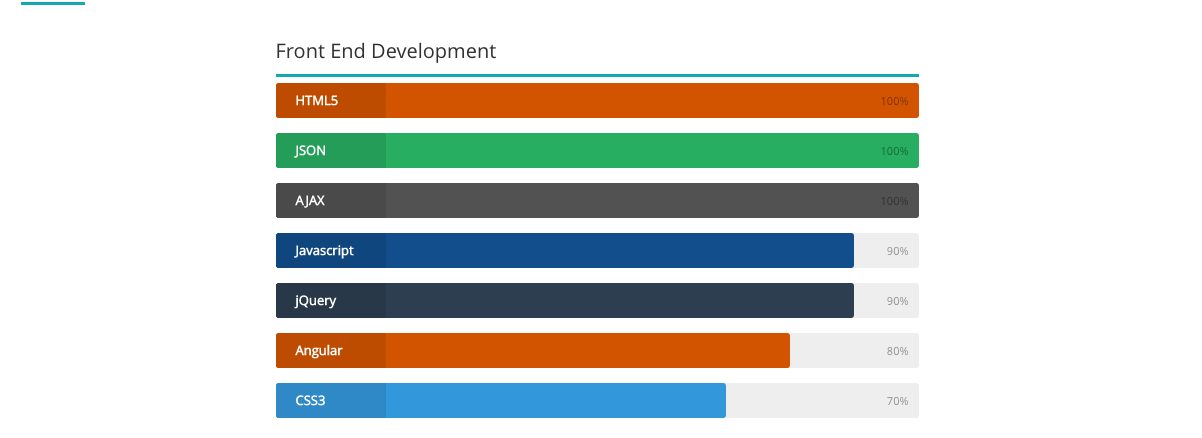
To see a working example of the skill bars. Go to Example
The HTML
Use the following html where you would like to have a skill bar section added.
<!-- Use a heading to reveal the skills section -->
<a href="javascript:void(0)" class="reveal">Testing</a>
<div id="testing-skills" class="skills-box">
<!-- Skill to make a bar out of -->
<skillbar percent="100" color="239d57">
HTML5
</skillbar>
</div>
The Javascript
Include the following javascript in the $(document).ready
section.
$(document).ready(function($) {
/*----------------------------------------------------*/
/* skill bars
------------------------------------------------------*/
/* Animate skillBars in the next section - Deac Karns */
$(".reveal").click(function(){
$(this).next().fadeIn();
$(this).next().find('.skillbar').each(function(){
$(this).find('.skillbar-bar').animate({
width:$(this).attr('data-percent')
},1500);
});
});
/* SkillBar directive - Transclude text body - Deac Karns */
$('skillbar').each(function(){
var percent = $(this).attr('percent');
var text = $(this).text();
var color = $(this).attr('color');
var html =
'<div class="skillbar clearfix " data-percent="'+percent+'%">'+
'<div class="skillbar-title" style="background: #'+color+';"><span>'+text+'</span></div>'+
'<div class="skillbar-bar" style="background: #'+color+';"></div>'+
'<div class="skill-bar-percent">'+percent+'%</div>'+
'</div>'
$(this).html(html);
});
The CSS
Here is the CSS to style the bars.
.skillbar {
position:relative;
display:block;
margin-bottom:15px;
width:100%;
background:#eee;
height:35px;
border-radius:3px;
-moz-border-radius:3px;
-webkit-border-radius:3px;
-webkit-transition:0.4s linear;
-moz-transition:0.4s linear;
-ms-transition:0.4s linear;
-o-transition:0.4s linear;
transition:0.4s linear;
-webkit-transition-property:width, background-color;
-moz-transition-property:width, background-color;
-ms-transition-property:width, background-color;
-o-transition-property:width, background-color;
transition-property:width, background-color;
}
.skillbar-title {
position:absolute;
top:0;
left:0;
width:110px;
font-weight:bold;
font-size:13px;
color:#ffffff;
background:#6adcfa;
-webkit-border-top-left-radius:3px;
-webkit-border-bottom-left-radius:4px;
-moz-border-radius-topleft:3px;
-moz-border-radius-bottomleft:3px;
border-top-left-radius:3px;
border-bottom-left-radius:3px;
}
.skillbar-title span {
display:block;
background:rgba(0, 0, 0, 0.1);
padding:0 20px;
height:35px;
line-height:35px;
-webkit-border-top-left-radius:3px;
-webkit-border-bottom-left-radius:3px;
-moz-border-radius-topleft:3px;
-moz-border-radius-bottomleft:3px;
border-top-left-radius:3px;
border-bottom-left-radius:3px;
}
.skillbar-bar {
height:35px;
width:0px;
background:#6adcfa;
border-radius:3px;
-moz-border-radius:3px;
-webkit-border-radius:3px;
}
.skill-bar-percent {
position:absolute;
right:10px;
top:0;
font-size:11px;
height:35px;
line-height:35px;
color:#ffffff;
color:rgba(0, 0, 0, 0.4);
}